JavaScript: Array Manipulation
-
Eric Stanley
- December 30, 2019
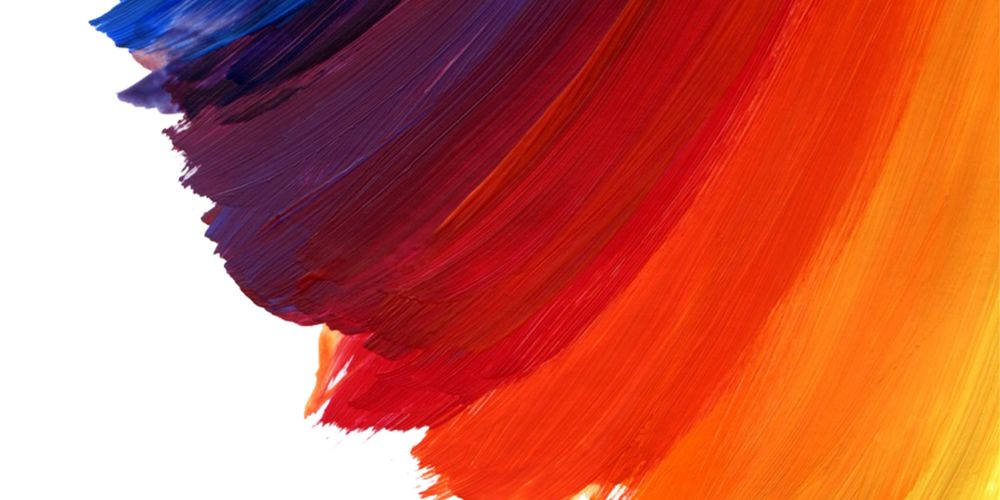
JavaScript offers different ways to manipulate arrays. However, there are methods that changes the original array and other methods that don’t. The methods that changes original array is called MUTATION. Let’s take a look at most common methods to manipulate arrays in JavaScript
Now, that we know that there are methods that mutates the original array, it’s considered best practice to assign the array to const
, if you know that the array that you use is not going to get mutated. It’s wise to use let
otherwise. It is not a mandate that you need to use const
to declare an array that will not be mutated, however, it’s easy for your colleague to understand that the array which is declared as const
is not going to get changed anywhere in the code; which is why it’s considered best practice
As usual, if you wanna try the examples in this post, I recommend codepen.io, but you are free to make your own choice.
The most common interactions that we usually have with arrays are
- Add item to array
- Remove item from array
- Update and item in the array
All the above mentioned actions can be done by both mutating and non-mutating methods. Instead of explaining each of the methods subjectively, I felt it would be better understood with a table. Here we go!
testArray: [a, b, c, d, e, f, g, h, i];
Code | Original Array | Processed Array | Returned Val | Is Mutated | Action | Method |
---|---|---|---|---|---|---|
testArray.push(‘j’) | [a,b,c,d,e,f,g,h,i] | [a,b,c,d,e,f,g,h,i,j] | 10 | true | add | push() |
testArray.unshift(‘z’) | [a,b,c,d,e,f,g,h,i,j] | [z,a,b,c,d,e,f,g,h,i,j] | 11 | true | add | unshift() |
testArray.concat(‘k’) | [z,a,b,c,d,e,f,g,h,i,j] | [z,a,b,c,d,e,f,g,h,i,j] | [z,a,b,c,d,e,f,g,h,i,j,k] | false | add | concat() |
[‘y’, …testArray, ‘l’] | [z,a,b,c,d,e,f,g,h,i,j] | [z,a,b,c,d,e,f,g,h,i,j] | [y,z,a,b,c,d,e,f,g,h,i,j,l] | false | add | … |
testArray.pop() | [z,a,b,c,d,e,f,g,h,i,j] | [z,a,b,c,d,e,f,g,h,i] | j | true | remove | pop() |
testArray.shift() | [z,a,b,c,d,e,f,g,h,i] | [a,b,c,d,e,f,g,h,i] | z | true | remove | shift() |
testArray.splice(0, 2) | [a,b,c,d,e,f,g,h,i] | [c,d,e,f,g,h,i] | [a,b] | true | remove | splice() |
testArray.filter(a => a!== ‘c’) | [c,d,e,f,g,h,i] | [c,d,e,f,g,h,i] | [d,e,f,g,h,i] | false | remove | filter() |
testArray.slice(1, 6) | [c,d,e,f,g,h,i] | [c,d,e,f,g,h,i] | [d,e,f,g,h] | false | remove | slice() |
testArray.slice(2) | [c,d,e,f,g,h,i] | [c,d,e,f,g,h,i] | [e,f,g,h,i] | false | remove | slice() |
testArray.splice(2, 1,30, 31) | [c,d,e,f,g,h,i] | [c,d,30,31,f,g,h,i] | [e] | true | update | splice() |
testArray.map(x => x ===‘d’ ? 29 : x) | [c,d,30,31,f,g,h,i] | [c,d,30,31,f,g,h,i] | [c,29,30,31,f,g,h,i] | false | update | map() |
I hope the above table is self-explanatory, however if you need to check the values of the testArray
with real code, feel free to visit programmatic output where the exact same table is derived programmatically.